Getting Started with Webhooks: Part 2 - Webhook Clients
A comprehensive two-part guide to understanding webhooks; this part focuses on webhook clients.
In the first part of this series, we covered webhook servers, what they are, how they work, and subsequently deployed a simple webhook server using Node.js and Express on Railway. Today, in the second part, we'll focus on webhook clients, what they are, how they interact with webhook servers, and then deploy a simple webhook client using Streamlit on Railway.
Getting Started with Webhook Clients
A webhook client is the system that sends webhook requests when certain events occur. It constructs an HTTP request and sends it to the webhook server's registered URL. Here are the general steps to be followed when setting up a webhook client:
- Identify relevant events: Determine which events should trigger webhook requests.
- Generate webhook payloads: When an event occurs, create a serialised form-encoded JSON payload with relevant data.
- Send webhook requests: Construct an HTTP POST request with the JSON payload and send it to the webhook server's URL.
In this post, we'll create and deploy a simple webhook client using Streamlit. Here is the sample client-side code; you can find the GitHub repository here.
import json, requests
import streamlit as st
st.subheader("Webhook Client")
# Default URL points to local server
url = st.text_input("Webhook URL", "http://localhost:3000/webhook-1")
payload = st.text_area("JSON Payload", "{}", height=200)
headers = {"Content-Type": "application/json"}
if st.button("Submit"):
if not url.strip():
st.error("Please provide the Webhook URL.")
else:
try:
# Validate JSON
parsed_payload = json.loads(payload)
response = requests.post(url, json=parsed_payload, headers=headers)
st.success(f"Status Code: {response.status_code}")
st.json(response.json() if response.headers.get("Content-Type") == "application/json" else response.text)
except json.JSONDecodeError:
st.error("Invalid JSON payload.")
except Exception as e:
st.error(f"Error: {str(e)}")
Deploy a Webhook Client with Streamlit on Railway
Railway is a modern app hosting platform that makes it easy to deploy production-ready apps quickly. Railway offers persistent database services for PostgreSQL, MySQL, MongoDB, and Redis, as well as application services with a GitHub repository as the deployment source. For the latter, Railway can automatically determine the application runtime and deploy the service. Since we are just testing the waters, Railway's free tier should be sufficient to host the service.
Sign up for an account with Railway using GitHub, and click Authorize Railway App
when redirected. Review and agree to Railway's Terms of Service and Fair Use Policy if prompted. Launch the Webhooks one-click starter template (or click the button below) to deploy the webhook client and server instantly on Railway.
We're deploying two services here - one each for the webhook client and server. For each, you'll be given an opportunity to change the default repository name and set it private, if you'd like. Accept the defaults and click Deploy
; the deployment will kick off immediately.
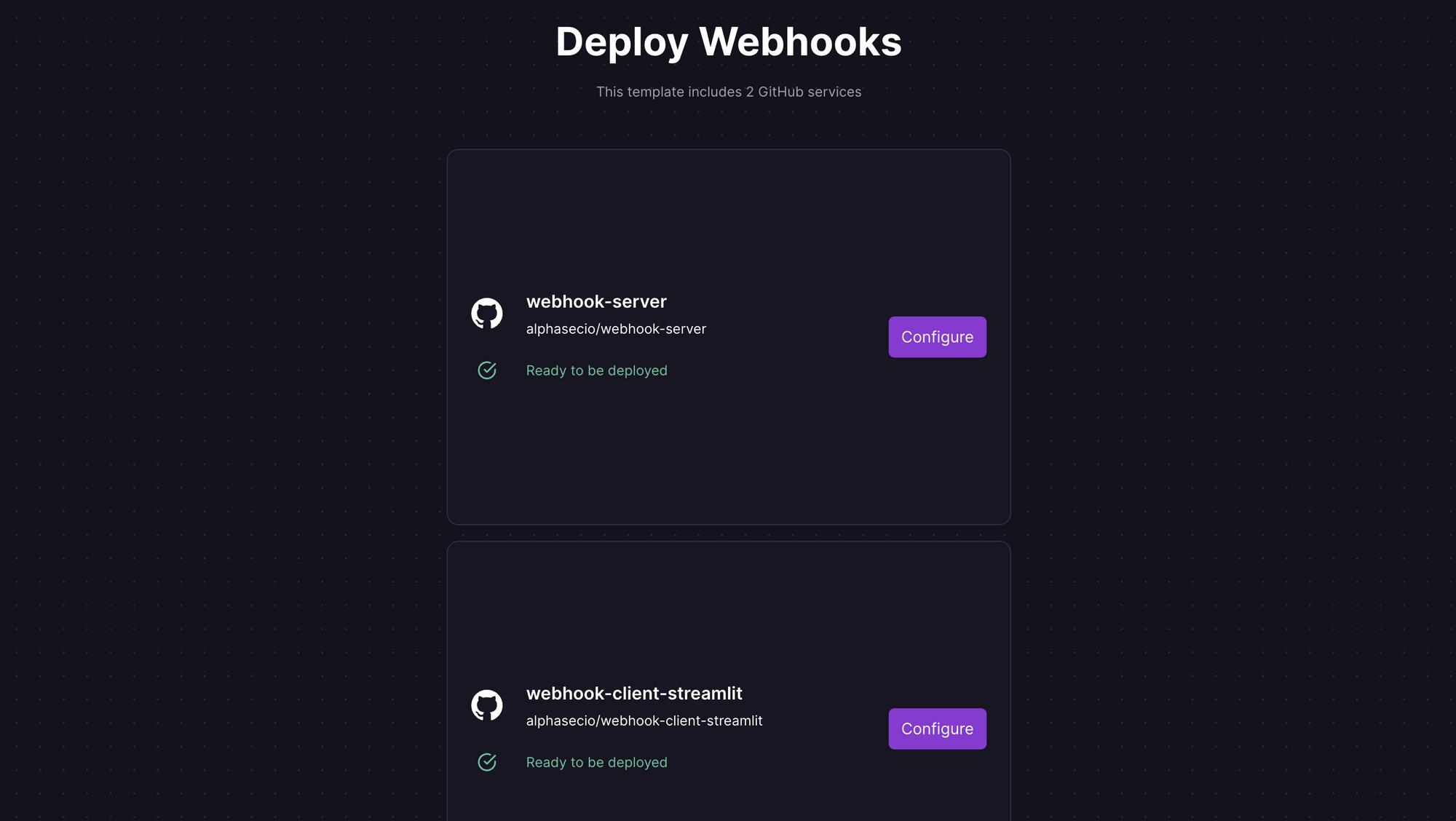
Once the deployment completes, the webhook client will be available at a default xxx.up.railway.app
domain - launch this URL to access the app. If you are interested in setting up a custom domain, I covered it at length in a previous post - see the final section here.
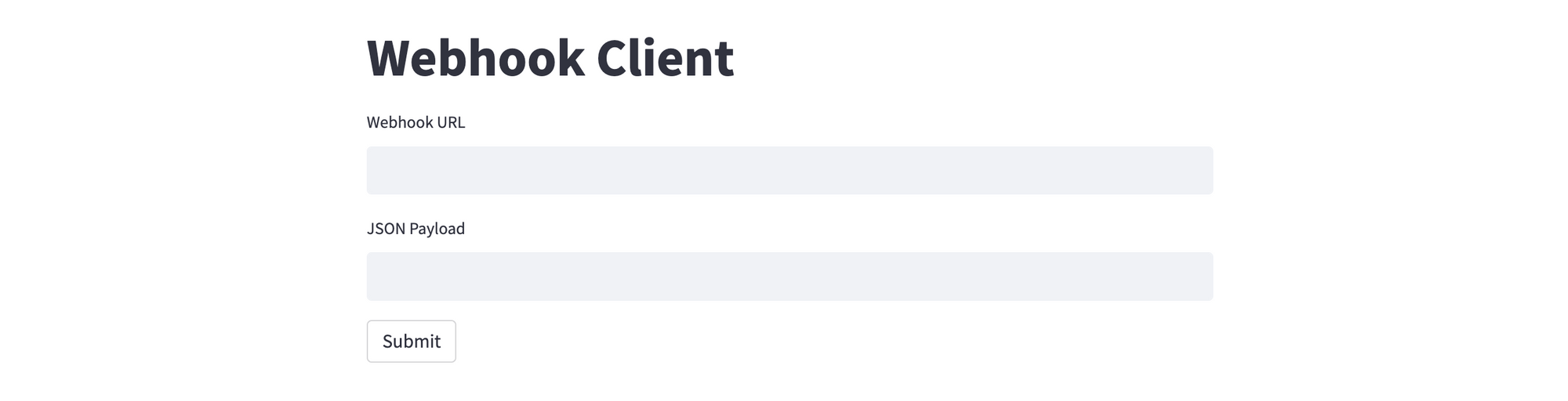
Provide the webhook server endpoint URL e.g. https://webhook-server.up.railway.app/webhook-1
and a sample JSON payload, and click Submit
.
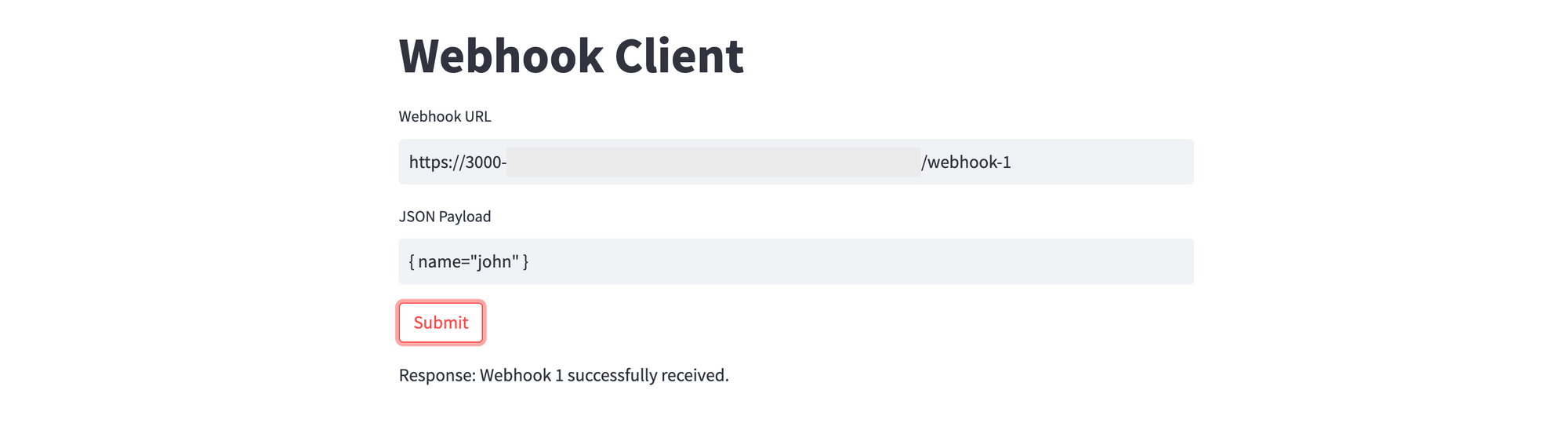
If your deployment was successful, an HTTP POST request will be made to the endpoint /webhook-1
to elicit the response Webhook 1 successfully received.
That's it! Of course, please bear in mind this is an extremely simplistic example, and should not be used as-is in any production capacity without appropriate security measures.
Security, Reliability and Other Considerations
Now that we've explored the concept of webhooks, common use cases, differences between webhook servers and clients, and deployed them, let's review the security, reliability, and other deployment considerations.
- Security: Webhooks can expose sensitive data if not properly secured. Use HTTPS, authenticate requests, and validate timestamps/payloads to enhance security. Mutual TLS authentication can also be used for added security.
- Data privacy and compliance: Review legal and regulatory requirements around data handling and sharing, especially PII data. The use of webhooks must comply with applicable regulations to avoid fines and legal liabilities.
- Reliability: Webhook requests can fail due to network issues or server downtime. Implement error handling and retry mechanisms, fail gracefully, and log requests to improve reliability.
- Payload size: Large payloads can cause performance issues. Optimize payloads by including only necessary data. Generally, webhooks are good for lightweight, specific actions and payloads.
- Rate limiting: Some webhook servers may impose rate limits. Be aware of these limits and adjust your webhook client accordingly.
- Monitoring: Monitor your webhook infrastructure to detect and troubleshoot issues quickly.
- Finally, not all applications support webhook integrations. Review the available APIs to determine the best method for communications.