Build an Interactive Python Web App with Streamlit
A brief guide to building and deploying an interactive Python web app on Streamlit Cloud.
What is Streamlit?
Streamlit is an open-source Python library that allows you to create and share interactive web apps and data visualisations in Python with ease. You create web apps using Python code, but with powerful add-on capabilities by Streamlit. It includes built-in support for several data visualisation libraries like matplotlib, pandas, and plotly, making it easy to create interactive charts and graphs that update in real-time based on user input. Streamlit is quite popular among data scientists, machine learning (ML) engineers, and developers looking to create and share interactive web apps with their audience.
Due to its rising popularity in the ML and data science communities, Streamlit was acquired by Snowflake in March 2022. Just before they got acquired, Streamlit announced the launch of their cloud-hosted service. Streamlit Cloud is a platform that enables you to deploy, manage, and share Streamlit apps easily. It provides analytics and usage metrics for your app, which also updates instantly when you push code changes to your connected git repository. The base tier allows one private app and unlimited public apps to be hosted for free. In this guide, we'll deploy a simple stock quote app on Streamlit Cloud, as well as Railway.
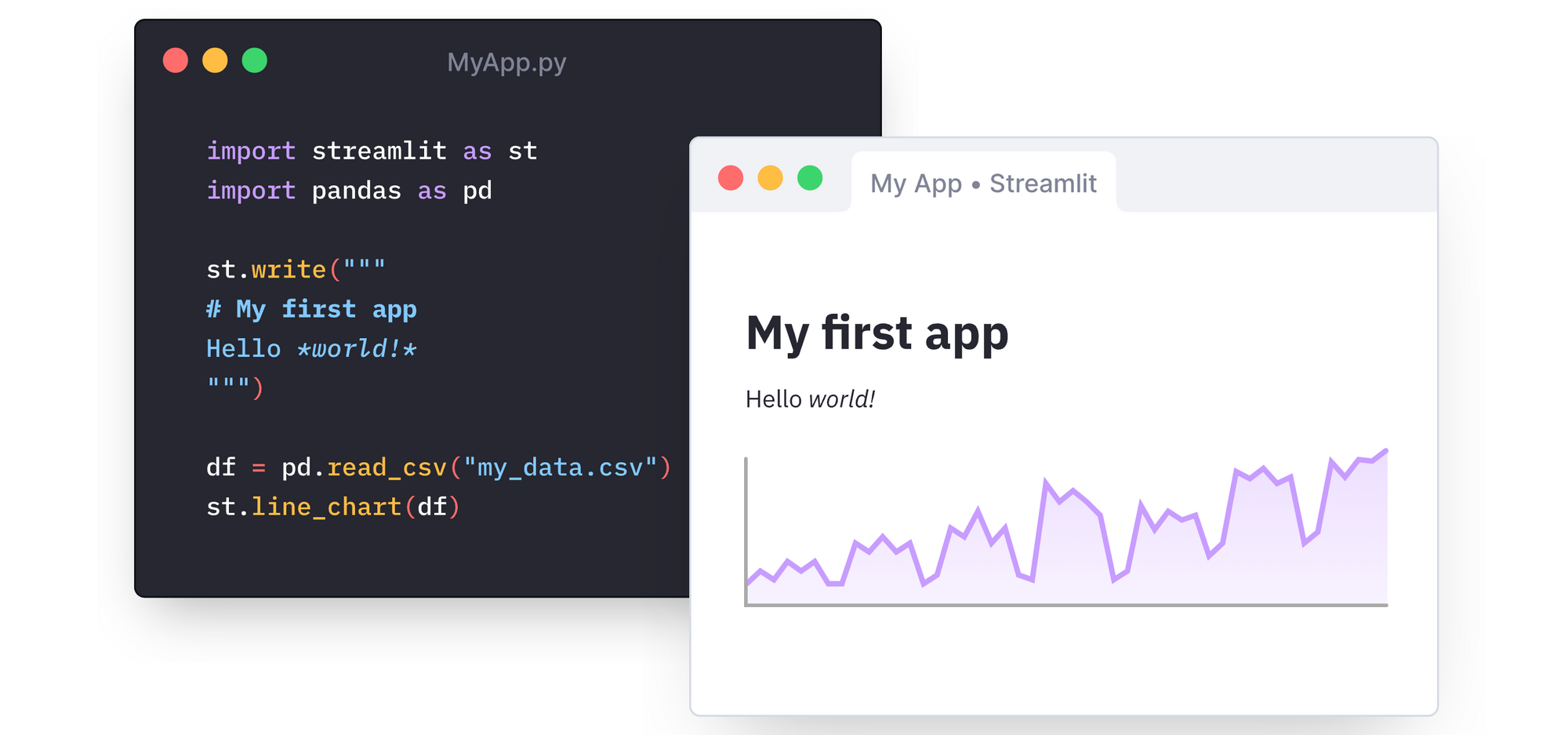
Deploy a Simple Python App on Streamlit
This tutorial uses the Polygon API to retrieve stock quote information. Sign up for a free individual user account with Polygon, and generate an API token. If you are familiar with, or have access to, other financial data providers, feel free to use your preferred service instead.
Getting started with Streamlit is quite easy - create an account on Streamlit Cloud, fork my sample app GitHub repository, connect your Streamlit account to GitHub, and deploy your app using the forked repository in a few clicks. In fact, if you just want to create a simple Hello World
app, all you need are two files - streamlit_app.py
and requirements.txt
. The former would have the following lines of code, while the latter would just include streamlit
as a dependency.
import streamlit as st
st.write('Hello World!')
I'll assume that you have forked my repository. I'm using the polygon-api-client
library to retrieve the stock data. Here's my streamlit_app.py
file.
import os, streamlit as st
from polygon import RESTClient
# Set up the Streamlit app
st.subheader("Stocks App")
symbol = st.text_input("Enter a stock symbol", "AAPL")
with st.sidebar:
polygon_api_key = st.text_input("Polygon API Key", type="password")
# Authenticate with the Polygon API
client = RESTClient(polygon_api_key)
col1, col2 = st.columns(2)
if col1.button("Get Details"):
if not polygon_api_key.strip() or not symbol.strip():
st.error("Please provide the missing fields.")
else:
try:
details = client.get_ticker_details(symbol)
st.success(f"Ticker: {details.ticker}\n\n"
f"Company Address: {details.address}\n\n"
f"Market Cap: {details.market_cap}")
except Exception as e:
st.exception(f"Exception: {e}")
if col2.button("Get Quote"):
if not polygon_api_key.strip() or not symbol.strip():
st.error("Please provide the missing fields.")
else:
try:
aggs = client.get_previous_close_agg(symbol)
for agg in aggs:
st.success(f"Ticker: {agg.ticker}\n\n"
f"Close: {agg.close}\n\n"
f"High: {agg.high}\n\n"
f"Low: {agg.low}\n\n"
f"Open: {agg.open}\n\n"
f"Volume: {agg.volume}")
except Exception as e:
st.exception(f"Exception: {e}")
Connect to your GitHub account, and authorise access to Streamlit. From your Streamlit Cloud account, click New app
to create a Streamlit app.
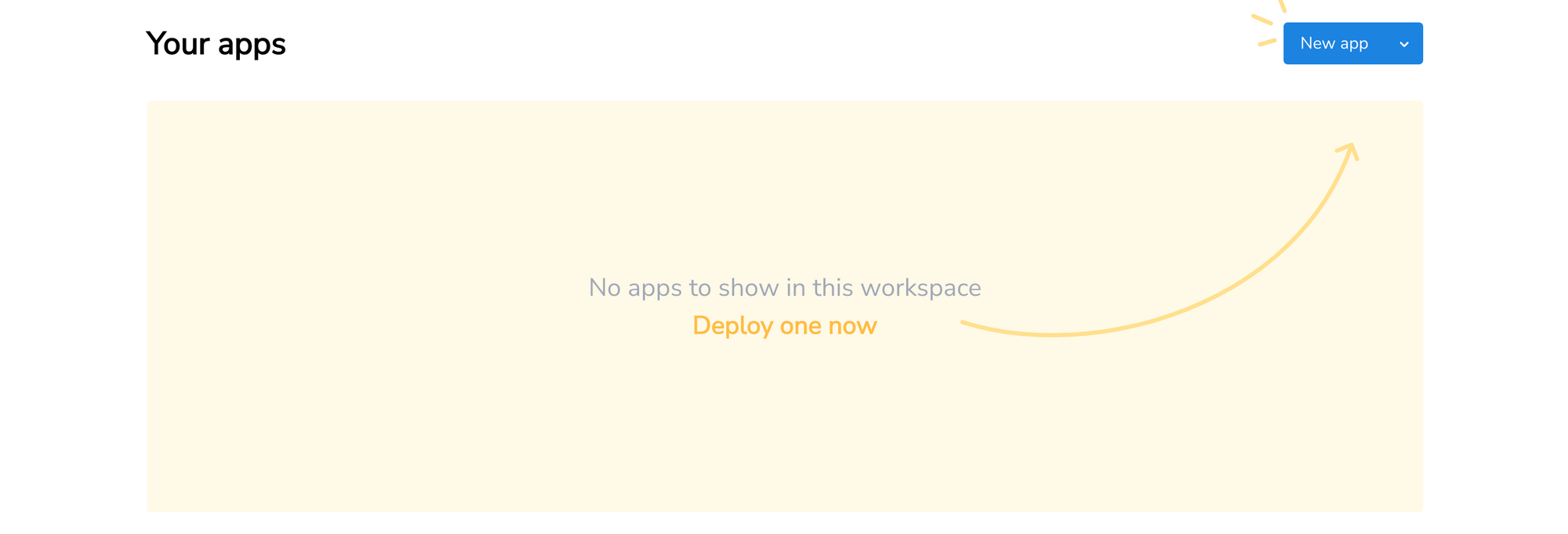
Select the main branch of the forked repository, and the default streamlit_app.py
main file, and click Deploy
.
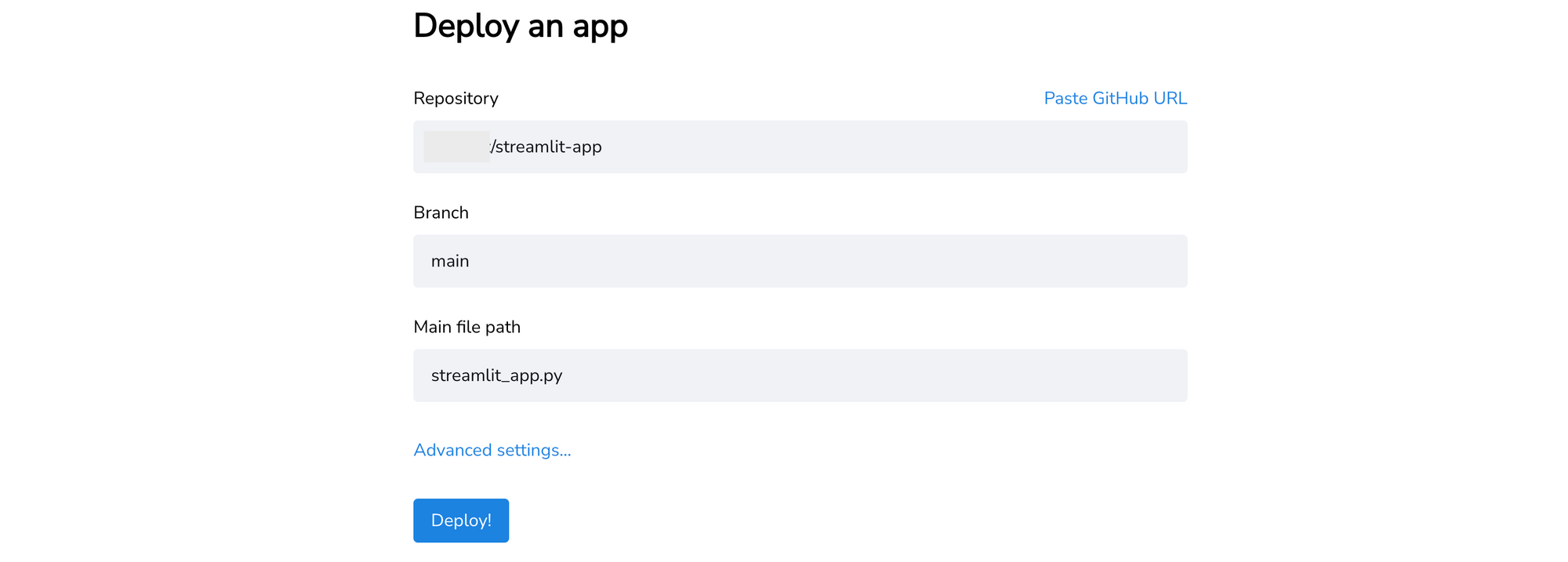
While the app is building, head over to Settings
and add a POLYGON_API_KEY
environment variable (instead of the TRADIER_TOKEN
variable displayed in the screenshot below) along with the corresponding value as an encrypted secret. You can also click the Advanced settings
option in the previous screen and specify the environment variable.
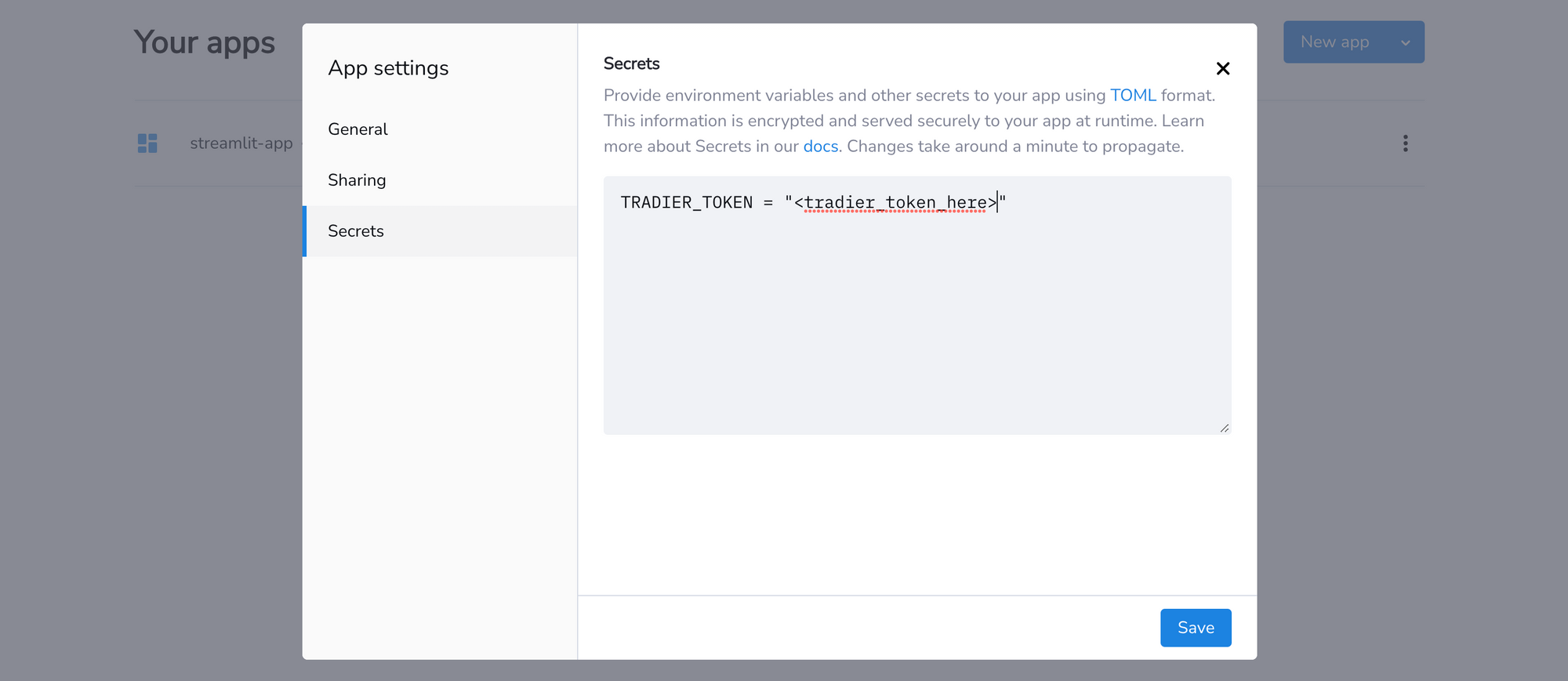
You may see the Your app is in the oven
message for a while, but your app should get deployed to a ***.streamlit.app
domain shortly. Specify the stock symbol (e.g. AAPL
) and click Get Quote
to retrieve the stock quote information. Voila! You have now deployed a fairly simple yet interactive app using Streamlit.
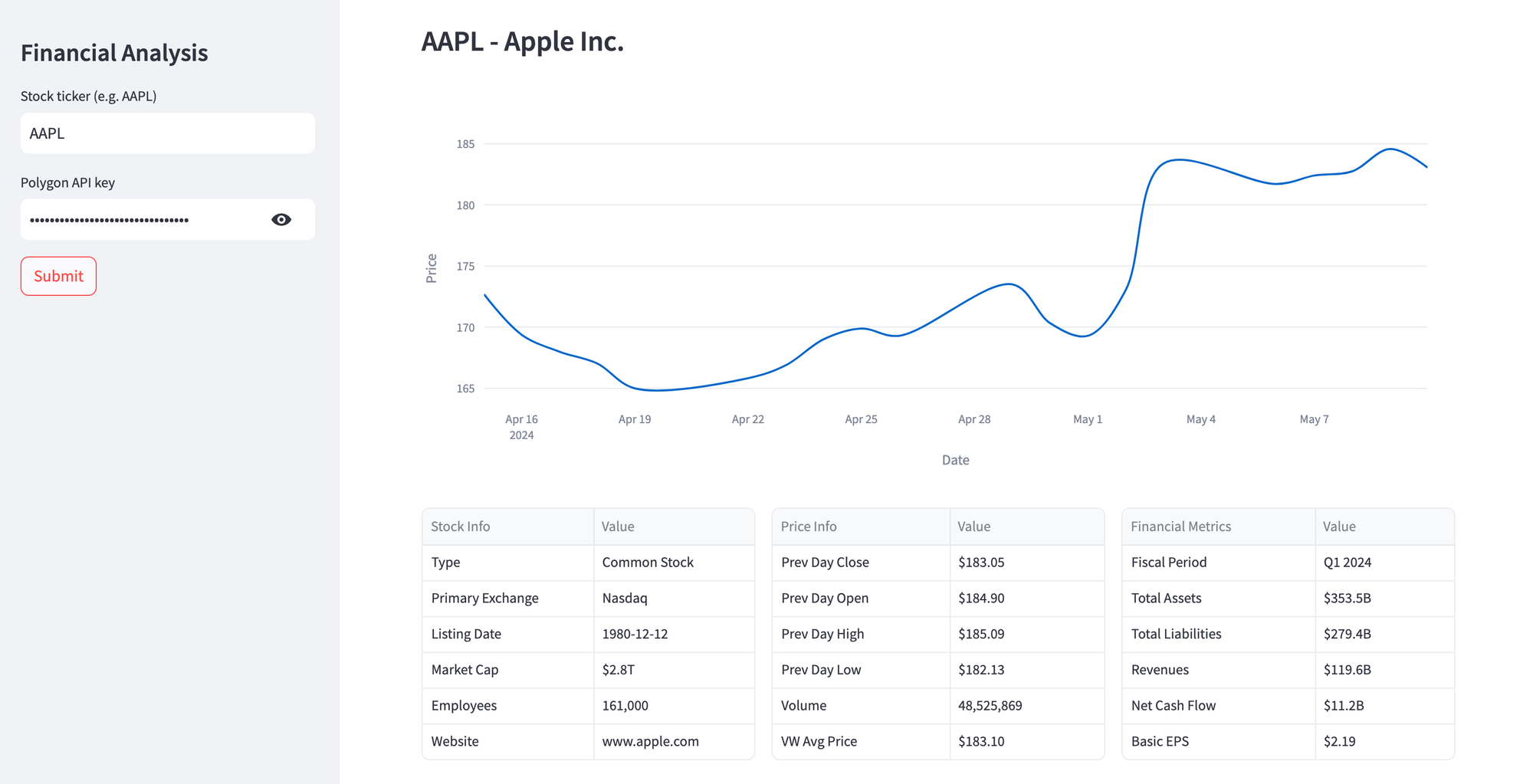
Instead of Streamlit Cloud, you can also deploy the app to other web hosting platforms of your choice. In the next section, I'll deploy the same app on Railway.
Deploy the Streamlit App on Railway
Railway is a modern app hosting platform that makes it easy to deploy production-ready apps quickly. Sign up for an account using GitHub, and click Authorize Railway App
when redirected. Review and agree to Railway's Terms of Service and Fair Use Policy if prompted. Launch the Streamlit Apps one-click starter template (or click the button below) to deploy the app instantly on Railway.
This template also deploys a Streamlit app that uses the Yahoo Finance API for stock ticker information - see this post for more details. Accept the defaults and click Deploy
; the deployment will kick off immediately.
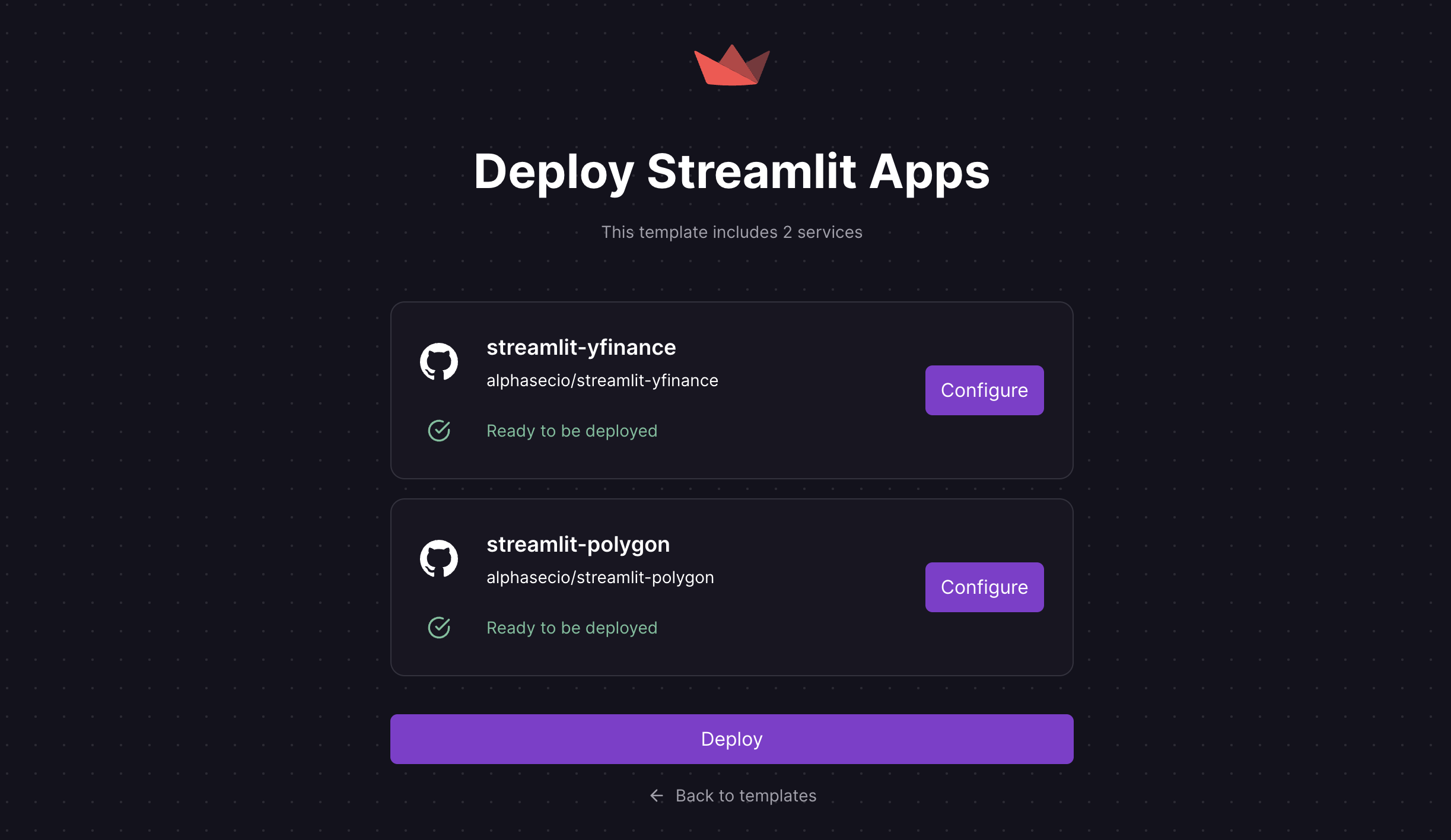
Once the deployment completes, the Streamlit apps will be available at a default xxx.up.railway.app
domain - launch this URL to access the app. If you are interested in setting up a custom domain, I covered it at length in a previous post - see the final section here.
Retrieve Stock Information using Yahoo Finance API
If you want to try the Yahoo Finance API instead of Polygon API, see this repository for a sample Streamlit web app. Launch the same one-click starter template (or click the button below) to deploy the app instantly on Railway.